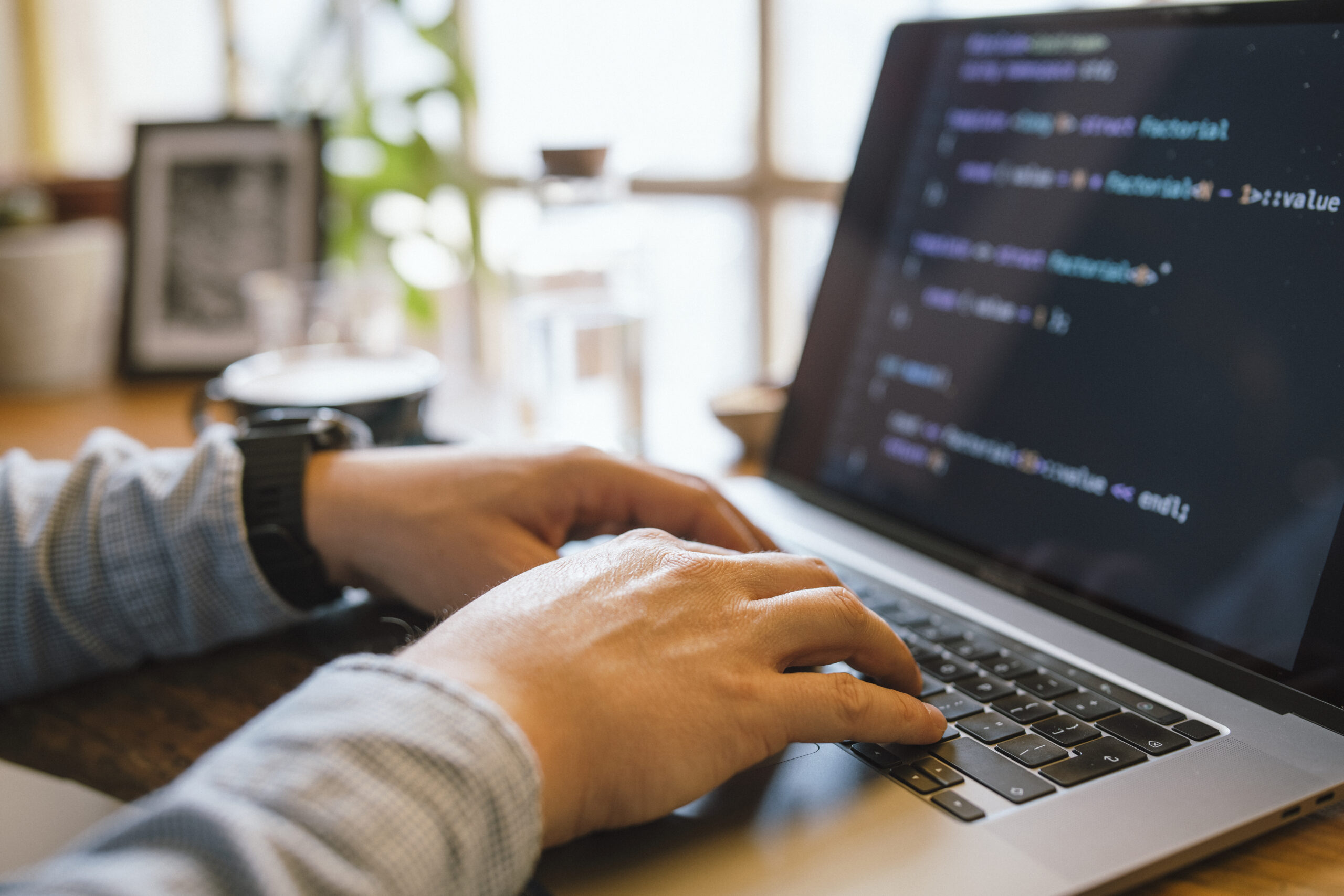
Debugging is Just about the most vital — nonetheless often ignored — capabilities in the developer’s toolkit. It isn't really pretty much correcting damaged code; it’s about understanding how and why things go Improper, and Mastering to Feel methodically to solve problems efficiently. No matter whether you are a starter or a seasoned developer, sharpening your debugging abilities can help save several hours of irritation and significantly boost your productivity. Allow me to share many approaches to help you developers level up their debugging game by me, Gustavo Woltmann.
Learn Your Applications
On the list of fastest approaches developers can elevate their debugging expertise is by mastering the resources they use every day. Though producing code is one particular Portion of development, recognizing tips on how to communicate with it successfully throughout execution is Similarly significant. Present day improvement environments come Geared up with effective debugging capabilities — but quite a few developers only scratch the area of what these instruments can do.
Acquire, by way of example, an Integrated Improvement Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources assist you to set breakpoints, inspect the worth of variables at runtime, phase through code line by line, and in many cases modify code within the fly. When used the right way, they Allow you to notice just how your code behaves throughout execution, and that is invaluable for monitoring down elusive bugs.
Browser developer tools, for example Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, observe network requests, look at authentic-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can flip discouraging UI issues into manageable jobs.
For backend or system-degree builders, tools like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Command more than managing procedures and memory administration. Understanding these instruments can have a steeper Mastering curve but pays off when debugging overall performance troubles, memory leaks, or segmentation faults.
Outside of your IDE or debugger, become cozy with Model Regulate units like Git to know code background, come across the exact instant bugs were introduced, and isolate problematic adjustments.
In the long run, mastering your tools indicates heading over and above default options and shortcuts — it’s about establishing an personal familiarity with your progress ecosystem so that when problems arise, you’re not misplaced at midnight. The higher you understand your equipment, the more time you'll be able to devote fixing the actual issue instead of fumbling via the process.
Reproduce the Problem
Probably the most critical — and often ignored — actions in efficient debugging is reproducing the problem. Before leaping in the code or generating guesses, developers need to produce a reliable setting or scenario where the bug reliably seems. With no reproducibility, fixing a bug becomes a activity of probability, usually leading to squandered time and fragile code adjustments.
The first step in reproducing a dilemma is gathering just as much context as you can. Inquire questions like: What steps resulted in the issue? Which atmosphere was it in — improvement, staging, or production? Are there any logs, screenshots, or mistake messages? The more depth you've got, the easier it will become to isolate the exact circumstances less than which the bug happens.
As you’ve collected enough data, attempt to recreate the situation in your local natural environment. This could indicate inputting exactly the same facts, simulating comparable consumer interactions, or mimicking system states. If The difficulty seems intermittently, take into account crafting automated assessments that replicate the sting circumstances or point out transitions involved. These exams don't just assist expose the challenge but will also stop regressions Sooner or later.
Sometimes, the issue may be natural environment-specific — it might transpire only on sure operating programs, browsers, or underneath particular configurations. Making use of instruments like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms might be instrumental in replicating these types of bugs.
Reproducing the problem isn’t only a phase — it’s a way of thinking. It necessitates patience, observation, along with a methodical strategy. But as soon as you can continuously recreate the bug, you happen to be by now midway to correcting it. Which has a reproducible state of affairs, you can use your debugging tools much more efficiently, examination likely fixes safely and securely, and converse far more Obviously using your crew or end users. It turns an abstract complaint into a concrete challenge — and that’s exactly where developers prosper.
Browse and Have an understanding of the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when one thing goes Improper. As opposed to seeing them as frustrating interruptions, builders really should study to deal with error messages as immediate communications with the technique. They usually tell you exactly what transpired, wherever it occurred, and occasionally even why it transpired — if you understand how to interpret them.
Commence by studying the information meticulously and in comprehensive. Lots of developers, especially when underneath time strain, glance at the 1st line and right away begin earning assumptions. But deeper in the mistake stack or logs might lie the legitimate root lead to. Don’t just copy and paste mistake messages into search engines like yahoo — read and fully grasp them initial.
Crack the error down into sections. Is it a syntax mistake, a runtime exception, or perhaps a logic error? Does it point to a certain file and line quantity? What module or purpose triggered it? These issues can manual your investigation and place you toward the accountable code.
It’s also practical to grasp the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java frequently comply with predictable styles, and Finding out to acknowledge these can dramatically increase your debugging procedure.
Some glitches are imprecise or generic, and in People conditions, it’s essential to examine the context where the mistake occurred. Check out similar log entries, input values, and recent alterations from the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger concerns and provide hints about probable bugs.
Finally, error messages aren't your enemies—they’re your guides. Finding out to interpret them effectively turns chaos into clarity, encouraging you pinpoint problems more quickly, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Sensibly
Logging is one of the most potent resources within a developer’s debugging toolkit. When utilised proficiently, it offers authentic-time insights into how an software behaves, supporting you fully grasp what’s occurring beneath the hood while not having to pause execution or phase throughout the code line by line.
A superb logging approach commences with figuring out what to log and at what stage. Widespread logging stages incorporate DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for in-depth diagnostic facts through progress, Data for typical situations (like prosperous start off-ups), WARN for possible issues that don’t crack the appliance, ERROR for actual complications, and Deadly once the system can’t go on.
Prevent flooding your logs with abnormal or irrelevant information. Too much logging can obscure vital messages and slow down your method. Deal with critical functions, state variations, enter/output values, and demanding decision details within your code.
Structure your log messages Obviously and consistently. Include things like context, including timestamps, ask for IDs, and function names, so it’s much easier to trace troubles in dispersed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re Primarily useful in output environments the place stepping through code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Finally, sensible logging is about harmony and clarity. Using a very well-thought-out logging technique, you could reduce the time it will require to identify problems, get further visibility into your programs, and Increase the General maintainability and dependability of your code.
Feel Similar to a Detective
Debugging is not just a specialized endeavor—it's a kind of investigation. To proficiently identify and deal with bugs, builders should technique the procedure just like a detective resolving a mystery. This attitude can help stop working complex problems into workable pieces and follow clues logically to uncover the root result in.
Start out by accumulating proof. Think about the indications of the issue: error messages, incorrect output, or overall performance problems. Much like a detective surveys a criminal offense scene, gather as much pertinent details as it is possible to with no jumping to conclusions. Use logs, examination circumstances, and consumer reviews to piece with each other a clear picture of what’s happening.
Next, variety hypotheses. Talk to you: What can be resulting in this habits? Have any alterations just lately been created for the codebase? Has this problem happened right before underneath related situations? The objective is to slender down options and discover prospective culprits.
Then, test your theories systematically. Seek to recreate the situation within a controlled natural environment. In case you suspect a specific functionality or part, isolate it and verify if The difficulty persists. Like a detective conducting interviews, ask your code issues and Allow the effects direct you closer to the reality.
Spend shut focus to small information. Bugs generally conceal during the minimum envisioned areas—similar to a missing semicolon, an off-by-a person error, or a race issue. Be thorough and client, resisting the urge to patch the issue with no fully knowledge it. Short-term fixes may well hide the true trouble, only for it to resurface later on.
Lastly, preserve notes on Anything you attempted and figured out. Equally as detectives log their investigations, documenting your debugging method can help save time for long term troubles and assistance Other individuals fully grasp your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical expertise, tactic complications methodically, and turn out to be simpler at uncovering concealed challenges in complicated techniques.
Produce Checks
Writing tests is one of the best solutions to help your debugging abilities and All round growth performance. Checks don't just help catch bugs early but also serve as a safety Internet that provides you self confidence when generating improvements towards your codebase. A perfectly-analyzed software is much easier to debug mainly because it helps you to pinpoint exactly where and when a problem occurs.
Get started with device checks, which deal with unique capabilities or modules. These smaller, isolated assessments can speedily reveal no matter whether a particular piece of logic is Operating as expected. Any time a exam fails, you promptly know wherever to seem, drastically lowering the time spent debugging. Device assessments are Specially valuable for catching regression bugs—troubles that reappear right after Formerly being fixed.
Future, combine integration assessments and finish-to-end checks into your workflow. These support make certain that various aspects of your application work alongside one another efficiently. They’re especially practical for catching bugs that arise in sophisticated systems with many elements or products and services interacting. If anything breaks, your tests can inform you which Portion of the pipeline unsuccessful and beneath what conditions.
Composing exams also forces you to definitely Feel critically regarding your code. To test a aspect appropriately, you'll need to be aware of its inputs, anticipated outputs, and edge scenarios. This degree of being familiar with By natural means potential customers to better code framework and much less bugs.
When debugging a problem, crafting a failing check that reproduces the bug is often a robust first step. After the take a look at fails constantly, you could concentrate on repairing the bug and check out your check move when The difficulty is resolved. This strategy makes certain that the same bug doesn’t return Later on.
Briefly, crafting tests turns debugging from a annoying guessing activity into a structured and predictable method—supporting you capture extra bugs, quicker and even more reliably.
Acquire Breaks
When debugging a tough issue, it’s simple to become immersed in the challenge—observing your monitor for several hours, trying Answer right after Remedy. But Among the most underrated debugging instruments is solely stepping absent. Having breaks allows you reset your intellect, reduce frustration, and often see the issue from the new standpoint.
If you're much too near the code for much too extensive, cognitive exhaustion sets in. You may perhaps get started overlooking noticeable faults or misreading code that you wrote just several hours before. During this point out, your brain turns into significantly less effective at issue-solving. A brief stroll, a coffee break, or simply switching to another undertaking for 10–15 minutes can refresh your focus. Lots of builders report locating the root of a problem when they've taken time and energy to disconnect, letting their subconscious work during the qualifications.
Breaks also aid stop burnout, Primarily through more time debugging sessions. Sitting down in front of a screen, mentally caught, is not just unproductive but also draining. Stepping absent enables you to return with renewed energy and also a clearer attitude. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
When you’re stuck, a very good guideline should be to set a timer—debug actively for forty five–60 minutes, then have a 5–ten minute split. Use that point to move all-around, stretch, or do a thing unrelated to code. It might sense counterintuitive, Particularly underneath tight deadlines, but it surely really contributes to faster and simpler debugging Ultimately.
In brief, getting breaks is not a sign of weak spot—it’s a sensible method. It offers your Mind Area to breathe, enhances your standpoint, and can help you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is a component of resolving it.
Learn From Every single Bug
Each individual bug you encounter is much more than just A brief setback—It is really an opportunity to expand being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or perhaps a deep architectural situation, each can instruct you something beneficial should you make time to replicate and review what went wrong.
Begin by asking your self several essential issues as soon as the bug is fixed: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with much better methods like unit testing, code critiques, or logging? The answers frequently reveal blind places in the workflow or understanding and help you build stronger coding patterns going ahead.
Documenting bugs can even be an outstanding practice. Hold a developer journal or keep a log where you Be aware down more info bugs you’ve encountered, the way you solved them, and Anything you figured out. After some time, you’ll start to see patterns—recurring issues or popular faults—which you could proactively keep away from.
In group environments, sharing what you've acquired from the bug along with your peers is usually Specially effective. Whether or not it’s via a Slack concept, a short generate-up, or a quick knowledge-sharing session, encouraging Other folks avoid the exact situation boosts group performance and cultivates a more powerful learning lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a few of the finest developers are certainly not the ones who produce ideal code, but people that continuously study from their errors.
In the long run, Every bug you correct provides a fresh layer towards your skill set. So future time you squash a bug, take a second to replicate—you’ll occur away a smarter, far more able developer due to it.
Summary
Improving upon your debugging abilities normally takes time, observe, and patience — even so the payoff is large. It makes you a more productive, self-assured, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become far better at That which you do.